7 Essential Angular 6 Recipes for Quick Projects

In the dynamic world of web development, having the right tools at your disposal can significantly boost your productivity and efficiency. Among these tools, Angular 6 stands out due to its robust features, component-based architecture, and comprehensive ecosystem for building single-page applications (SPAs). If you're embarking on or currently working on a project that requires quick turnaround, understanding some key Angular 6 recipes can be your shortcut to success. Here, we explore seven essential Angular 6 recipes that can help you develop projects rapidly.
1. Setting Up an Angular 6 Project

The first step to leveraging Angular 6 in your projects is setting up a new project. Here’s how you can do it:
- Ensure you have Node.js and npm installed. Then install the Angular CLI by running:
npm install -g @angular/cli
- Create a new project with:
ng new your-project-name
- Change into the project directory and start the development server:
cd your-project-name ng serve –open
This setup allows you to jumpstart your development process with an Angular environment already tailored for your project.
2. Creating Components Quickly

Components are the building blocks of Angular applications. Here’s how to quickly create and integrate a new component:
- Use the Angular CLI to generate a new component:
or for short:ng generate component component-name
ng g c component-name
Remember, efficient component creation saves time and helps in keeping your project's structure clean and organized.
3. Utilizing Angular Services

Services in Angular are designed for functionality that is not inherently tied to components. Here’s how to create a service:
- Generate a service using the CLI:
or for short:ng generate service service-name
ng g s service-name
Services are ideal for code reuse, especially for tasks like data sharing, state management, and HTTP requests.
4. Routing in Angular Applications

Angular’s router allows you to navigate between different views or components. Here’s a quick setup:
- Add the router module to your app module:
and define your routes in theimport { RouterModule, Routes } from ‘@angular/router’;
ngOnInit
method or in yourapp-routing.module.ts
.
Navigation is fundamental in SPAs, and Angular's router makes it straightforward.
5. Integrating Forms

Forms are a core interaction point in many applications. Angular 6 supports both Template-Driven and Reactive forms:
- For a Template-Driven Form, import the
FormsModule
, and usengModel
in your template. - For Reactive Forms, import
ReactiveFormsModule
, and useFormGroup
andFormControl
in your component.
Understanding which form to use can dramatically impact your project's usability and performance.
6. Implementing Authentication

Adding authentication to your Angular application can be streamlined with these steps:
- Set up a user model and a login component.
- Use JWT (JSON Web Tokens) for stateless authentication, or integrate with an auth service like Firebase or Auth0.
This ensures your application can secure endpoints and manage user sessions effectively.
7. Performance Optimization with AOT Compilation

Ahead-of-Time (AOT) compilation can significantly improve your application’s performance. Here’s how to configure it:
- Use the
–aot
flag when building your project:ng build –prod –aot
AOT compilation parses your templates and components at build time, which not only reduces the runtime costs but also enhances the overall user experience by reducing load times.
💡 Note: While these recipes are a great starting point, remember that every project has unique requirements. Tailor these techniques to suit your project's needs for optimal results.
In the journey of web development with Angular 6, these recipes serve as essential tools that can accelerate your project development cycle. From setting up a project to optimizing performance, each step is a building block towards creating efficient, responsive, and scalable applications. By leveraging these techniques, developers can focus more on creativity and functionality rather than on setup and basic configurations. These recipes not only streamline your workflow but also ensure that you're making the most out of what Angular 6 has to offer.
What are the advantages of using Angular 6 for web projects?

+
Angular 6 offers benefits like modular architecture for reusability, comprehensive tooling with CLI, powerful routing, a vast ecosystem, and performance optimizations through AOT compilation, making it ideal for complex SPAs.
How do I decide between Template-Driven and Reactive Forms in Angular?

+
Template-Driven forms are better for simple forms where most of the logic is in the template. Reactive Forms are preferred for complex forms where you need strong validation, form control, and dynamic updates in real-time.
What is AOT compilation and why should I use it?
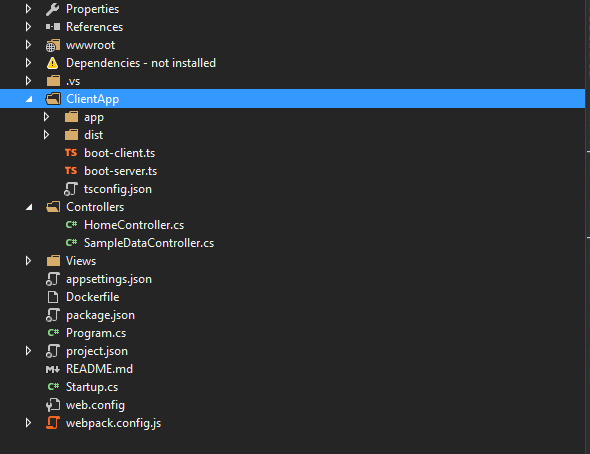
+
AOT (Ahead-of-Time) compilation in Angular converts templates and components into highly optimized JavaScript code at build time, reducing load time and enhancing performance by preventing additional parsing at runtime.
Related Terms:
- angular 6 recipe book