C++ Simplex Algorithm: Numerical Recipe Code Breakdown
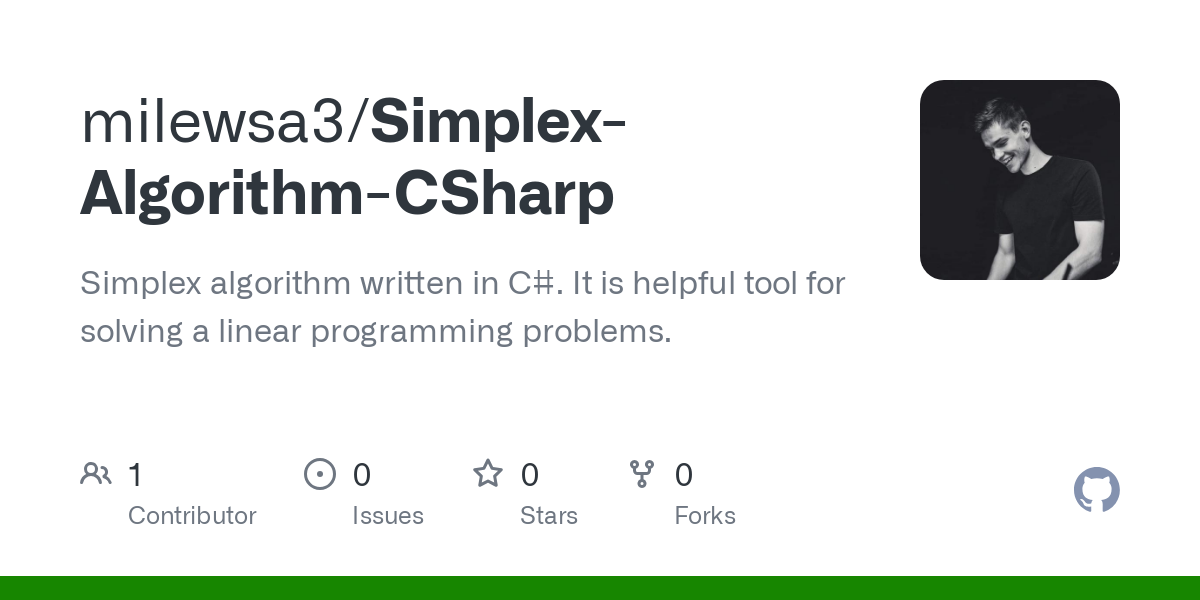
Whether you're a seasoned programmer or just stepping into the vast world of algorithmic complexity, understanding C++ Simplex Algorithm from Numerical Recipes offers a deep dive into optimization problems that have real-world applications. This blog post will guide you through the intricacies of the Simplex algorithm, a powerful linear programming method, breaking down its implementation in C++ as found in the iconic book, Numerical Recipes.
Understanding the Simplex Algorithm

The Simplex method is a popular technique used to solve linear programming problems, aiming to maximize or minimize a linear objective function subject to linear inequality and equality constraints. The approach involves moving through the feasible region's vertices, searching for the optimal solution step by step. Here's how:
- Start at an initial feasible point.
- Move in a direction that improves the objective function.
- Reevaluate at each vertex until an optimal solution is found or it's clear there is no better solution.

The C++ Code Structure

When examining the implementation in Numerical Recipes, we find a well-structured C++ code with these key components:
- Data structures: For managing the tableau, storing constraints, objective function coefficients, and other problem-specific data.
- Algorithm: The core logic for pivoting, which includes rules to identify entering and leaving variables, and handling special cases like degeneracy.
- Utilities: Helper functions for matrix manipulation, input/output, and error handling.
Key Functions in the Code

Function | Role |
---|---|
simplex() | Sets up the tableau and calls pivot functions to find the optimal solution. |
pivot() | Executes the pivot operation, selecting and executing the necessary row and column changes. |
enter_rule() | Determines which variable enters the basis. |
leave_rule() | Decides which variable leaves the basis. |
duality_check() | Verifies if the problem has an optimal solution or if it's unbounded. |

🔍 Note: Understanding these functions thoroughly helps in extending the algorithm for various LP problems.
Breaking Down the Code

Setup and Initialization

The code starts by setting up the tableau, which is a matrix representation of the LP problem. Here’s how it typically looks:
void simplex_setup(int m, int n, float **a, float *b, float *c) {
// Initialization code
}
Pivoting Mechanism

The core of the algorithm, pivoting, involves changing the basis:
- Finding the pivot element based on specific rules.
- Transforming the tableau to reflect the changes in the basis.
Error Handling and Special Cases

The Numerical Recipes code accounts for scenarios like degeneracy, infeasible, and unbounded solutions:
- Degeneracy: When more than one variable can leave the basis, special rules are applied.
- Infeasibility: When no solution exists within the constraints.
- Unboundedness: When the objective function can grow without bound.
⚠️ Note: Proper error handling enhances the robustness of the implementation, making it suitable for real-world applications.
Optimizing for Real-world Use

While the basic Simplex Algorithm is foundational, in practice, several optimizations and variations are commonly used:
- Revised Simplex: Reduces storage requirements by not storing the entire tableau.
- Phase I and Phase II: For problems with equality or mixed constraints, using two phases helps find a feasible solution first.
- Perturbation techniques: To handle numerical instability due to degeneracy or near-degeneracy.
- Interior Point Methods: An alternative to the Simplex method for very large problems.
Summarizing this journey through the C++ Simplex Algorithm from Numerical Recipes, we've seen how the code breaks down complex linear programming problems into manageable steps. This deep dive not only highlights the technical prowess required for such implementations but also underscores the elegance and efficiency of mathematical programming in solving real-life optimization challenges. Understanding these implementations can provide a solid foundation for tackling more complex problems in numerical optimization.
What are the main advantages of using the Simplex Algorithm?

+
The Simplex Algorithm is particularly efficient for problems where a feasible solution is known or can be found quickly, and the solution space is relatively small. Its advantages include simplicity, systematic approach, and the ability to find an optimal solution in a finite number of steps when such a solution exists.
How does degeneracy affect the Simplex Algorithm?

+
Degeneracy occurs when a pivot operation does not improve the objective function, potentially causing the algorithm to cycle through the same solutions. Techniques like lexicographic rules or perturbation methods are used to deal with this issue effectively.
Can the Simplex Algorithm handle non-linear programming?

+
Traditional Simplex is designed for linear programming problems. However, extensions like quadratic programming can sometimes be solved using variants of the Simplex Algorithm, or other optimization techniques like interior point methods are better suited for non-linear problems.
Related Terms:
- Simplex algorithm explained
- Simplex algorithm pseudocode
- Simplex method in C