5 C++ Coding Recipes to Boost Efficiency
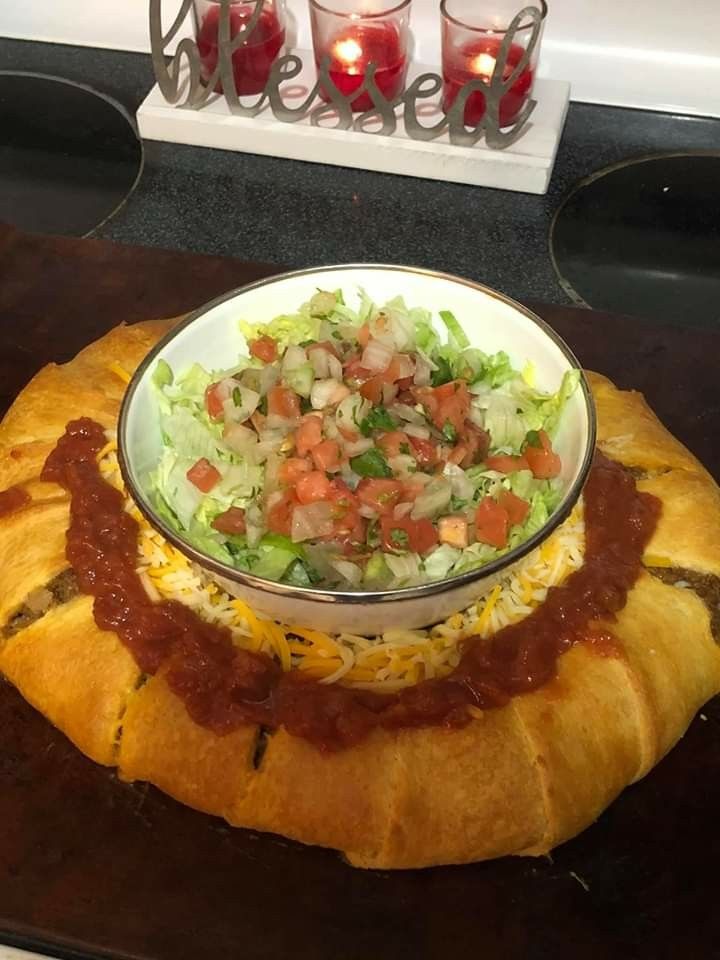
C++ is celebrated for its high performance and control over system resources, making it a top choice for applications where efficiency is paramount. Whether you're delving into game development, system programming, or any other high-demanding computational tasks, optimizing your code for performance can drastically improve the responsiveness and efficiency of your applications. Here, we'll explore five coding recipes tailored for C++ that promise to enhance your programming efficiency:
1. Using Move Semantics for Efficient Resource Management

Move semantics, introduced in C++11, enable the transfer of resources from one object to another without copying. This approach is particularly useful when dealing with large objects, reducing the overhead of resource copying operations:
- Function argument passing: Use rvalue references (&&) to move resources efficiently instead of copying.
- Return value optimization: Let the compiler optimize the return values of functions, which can often involve move semantics implicitly.
Example:
class HeavyObject {
std::vector data;
public:
HeavyObject() : data(1000, 0) {}
HeavyObject(HeavyObject&& other) : data(std::move(other.data)) {} // Move constructor
HeavyObject& operator=(HeavyObject&& other) {
data = std::move(other.data); // Move assignment operator
return *this;
}
// other members
};
HeavyObject func() {
return HeavyObject(); // The return value could be moved instead of copied
}
int main() {
HeavyObject obj = func(); // Move semantics should apply here
return 0;
}
💡 Note: Using move semantics not only saves resources but also reduces the runtime, especially with complex object manipulation.
2. Leveraging Auto and Lambda for Cleaner Code

The introduction of auto
and lambda functions has made C++ programming more expressive and less verbose:
- Auto: Automatically deduces the type of variable from its initializer.
- Lambda: Allows defining small anonymous functions at the point of usage.
Example:
#include
#include int main() { std::vector
nums = {3, 1, 4, 1, 5}; std::for_each(nums.begin(), nums.end(), { std::cout << n << std::endl; }); auto res = std::find_if(nums.begin(), nums.end(), [](auto n) { return n > 3; }); return 0;
}
3. Employing Smart Pointers for Memory Management

Memory leaks and resource management errors can be mitigated with the use of smart pointers. Here’s how you can leverage them for efficiency:
std::unique_ptr
for exclusive ownership.std::shared_ptr
when multiple pointers to the same object are needed.
Example:
#include
#include
class Resource {
public:
void doSomething() { std::cout << "Using Resource\n"; }
};
int main() {
std::unique_ptr resource1(new Resource());
resource1->doSomething();
std::shared_ptr shared1 = std::make_shared();
shared1->doSomething();
return 0;
}
4. Efficient Use of Standard Containers

C++ provides several standard library containers that are optimized for performance. Understanding their nuances can lead to significant efficiency gains:
Container | Use Case | Efficiency Note |
---|---|---|
std::vector |
Dynamic array where elements are added or removed | Fast access, but insertion/removal at the end can be expensive if resizing is required |
std::deque |
Dynamic sequence of elements with fast front and back insertions/deletions | Good for dynamic growth from both ends, but slower than vector for middle operations |
std::list |
Sequence where efficient insertion/deletion at any position is needed | Offers no constant-time random access |
std::set |
Maintains sorted unique elements | Fast lookup for existence |
std::map |
Associative array for key-value pairs | Efficient for searching and storing key-value pairs |

5. Embracing Concurrency with Modern C++

Modern C++ supports multi-threading and concurrency, which can dramatically increase performance by leveraging multiple cores. Here are some ways to utilize this:
- std::thread for creating and managing threads.
- std::mutex and std::lock_guard for synchronization.
Example:
#include
#include
void task(int id) {
std::cout << "Executing Task " << id << '\n';
}
int main() {
std::thread t1(task, 1);
std::thread t2(task, 2);
t1.join(); // Wait for t1 to finish
t2.join(); // Wait for t2 to finish
return 0;
}
⚠️ Note: Thread safety should always be considered when working with concurrency to prevent data races and ensure program integrity.
In summary, optimizing your C++ code for efficiency involves understanding and effectively using language features like move semantics, smart pointers, modern concurrency tools, and the appropriate standard library containers. These techniques not only improve the performance of your code but also make it more readable, maintainable, and less error-prone. By implementing these strategies, you're on your way to writing more efficient and robust C++ applications.
What is the advantage of using move semantics?

+
Move semantics allow for the efficient transfer of resources, reducing the need for expensive copy operations, thereby improving performance and memory usage.
Can smart pointers completely prevent memory leaks?

+
Smart pointers, like std::unique_ptr
and std::shared_ptr
, automate memory management which significantly reduces the chance of memory leaks if used correctly. However, incorrect usage or circular references with shared pointers can still lead to leaks.
How does auto help in writing cleaner code?

+
auto
allows the compiler to deduce the type of the variable automatically, reducing the likelihood of type-related errors and making code more concise.