Angular 6 Recipe Demo: Simplifying Development

Angular 6 Recipe Demo: Simplifying Development - Angular 6 has become a powerful tool for developers, especially in building complex web applications with its component-based architecture and TypeScript integration. Today, we'll delve into creating a simple recipe demo application that showcases how Angular can simplify development by integrating all the necessary features into one cohesive framework. This post will guide you through setting up, developing, and running a basic recipe application using Angular 6.
Setting Up Your Angular 6 Project

To start with Angular 6, you’ll need Node.js installed on your system. Here’s how you can set up your project:
- Install Angular CLI:
- Create a new Angular project:
- Change directory to the project folder:
- Run the development server:
npm install -g @angular/cli
ng new recipe-demo
cd recipe-demo
ng serve –open
⚙️ Note: If you encounter issues with dependencies, make sure your npm is up-to-date and consider using the –legacy-peer-deps flag if necessary.
Creating the Recipe Component

In Angular, components are fundamental. Let’s create a component for managing recipes:
- Generate a new component:
- Update the component template:
ng generate component recipe
Recipes

{{recipe.name}}
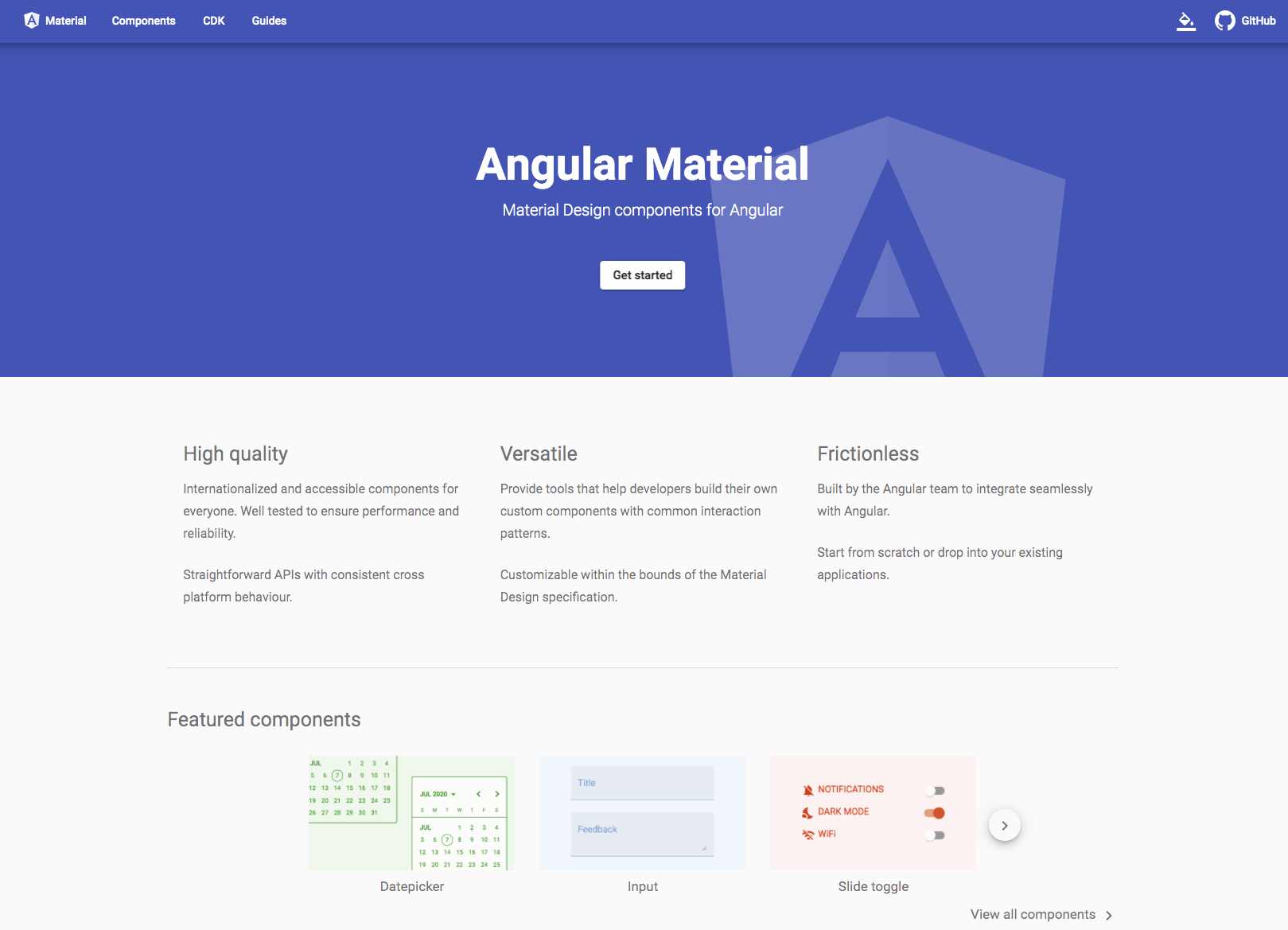
{{recipe.description}}
Ingredients: {{recipe.ingredients.join(‘, ‘)}}
// recipe.component.ts import { Component } from ‘@angular/core’;
@Component({ selector: ‘app-recipe’, templateUrl: ‘./recipe.component.html’, styleUrls: [‘./recipe.component.css’] }) export class RecipeComponent { recipes = [ { name: “Sushi”, description: “Delicious Japanese dish”, ingredients: [“Rice”, “Nori”, “Fish”] }, { name: “Pizza”, description: “A classic Italian delight”, ingredients: [“Dough”, “Cheese”, “Tomato Sauce”] } ]; }

Implementing Data Services

Data in Angular is often managed using services. Here’s how you can create and use a service for recipes:
- Generate a service:
- Modify the service to manage recipes:
ng generate service recipe
// recipe.service.ts import { Injectable } from ‘@angular/core’; import { Observable, of } from ‘rxjs’;
@Injectable({ providedIn: ‘root’ }) export class RecipeService { private recipes = [ // … same recipe array as above ];
getRecipes(): Observable
{ return of(this.recipes); }
addRecipe(recipe: any) { this.recipes.push(recipe); } }
// recipe.component.ts import { Component, OnInit } from ‘@angular/core’; import { RecipeService } from ‘./recipe.service’;
@Component({ selector: ‘app-recipe’, templateUrl: ‘./recipe.component.html’, styleUrls: [‘./recipe.component.css’] }) export class RecipeComponent implements OnInit { recipes: any[];
constructor(private recipeService: RecipeService) {}
ngOnInit() { this.recipeService.getRecipes().subscribe(recipes => this.recipes = recipes); } }
UI Enhancements with Forms

To allow users to add recipes, we’ll use Angular’s reactive forms:
- Install reactive forms if not already installed:
- Create a form within the RecipeComponent:
ng add @angular/forms
// recipe.component.ts import { Component, OnInit } from ‘@angular/core’; import { RecipeService } from ‘./recipe.service’; import { FormBuilder, FormGroup, Validators } from ‘@angular/forms’;
@Component({ selector: ‘app-recipe’, templateUrl: ‘./recipe.component.html’, styleUrls: [‘./recipe.component.css’] }) export class RecipeComponent implements OnInit { recipes: any[]; recipeForm: FormGroup;
constructor(private recipeService: RecipeService, private fb: FormBuilder) { this.recipeForm = this.fb.group({ name: [“, Validators.required], description: [”, Validators.required], ingredients: [“, Validators.required] }); }
ngOnInit() { this.recipeService.getRecipes().subscribe(recipes => this.recipes = recipes); }
onSubmit() { if (this.recipeForm.valid) { this.recipeService.addRecipe(this.recipeForm.value); this.recipeForm.reset(); } } }
…
Routing for Multi-page Application

To create a multi-page application, we’ll implement routing:
- Update app-routing.module.ts:
import { NgModule } from ‘@angular/core’; import { Routes, RouterModule } from ‘@angular/router’; import { RecipeComponent } from ‘./recipe/recipe.component’;
const routes: Routes = [ { path: “, component: RecipeComponent }, { path: ‘about’, component: AboutComponent } // You need to create this component ];
@NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
Through the development of this simple recipe application, we've explored several key features of Angular 6:
- Component Architecture - Building self-contained components for different parts of the application.
- Data Management - Using services to manage state across components.
- Reactive Forms - Handling user inputs in a more reactive manner.
- Routing - Navigating between different views of the application.
The simplicity and modularity of Angular 6 facilitate a development process that is not only efficient but also scalable. This setup provides a foundation on which you can build more complex applications, leveraging Angular's full suite of features to manage everything from simple lists to comprehensive, interactive user interfaces.
What is the benefit of using services in Angular?

+
Services in Angular help in separating the concerns of fetching, managing, and providing data or logic which can be shared across multiple components, enhancing code reuse and maintainability.
How do I start with Angular if I’m a beginner?
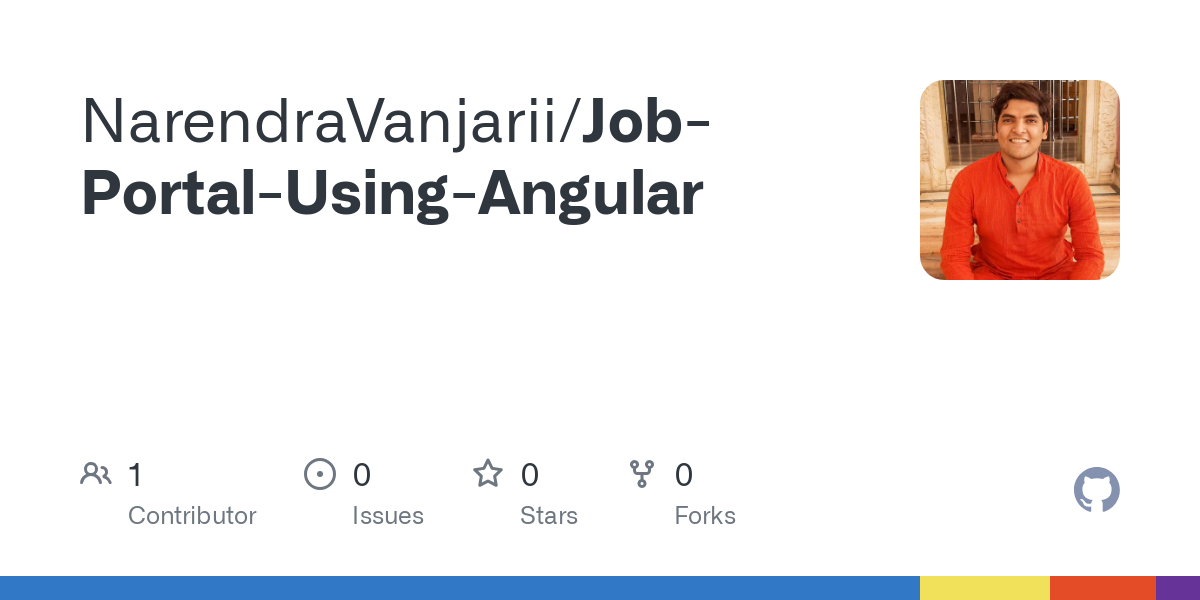
+
Begin with learning TypeScript, then move on to Angular fundamentals like components, modules, services, and templates. Tutorials and the official Angular documentation are great starting points.
Can I use Angular for mobile app development?
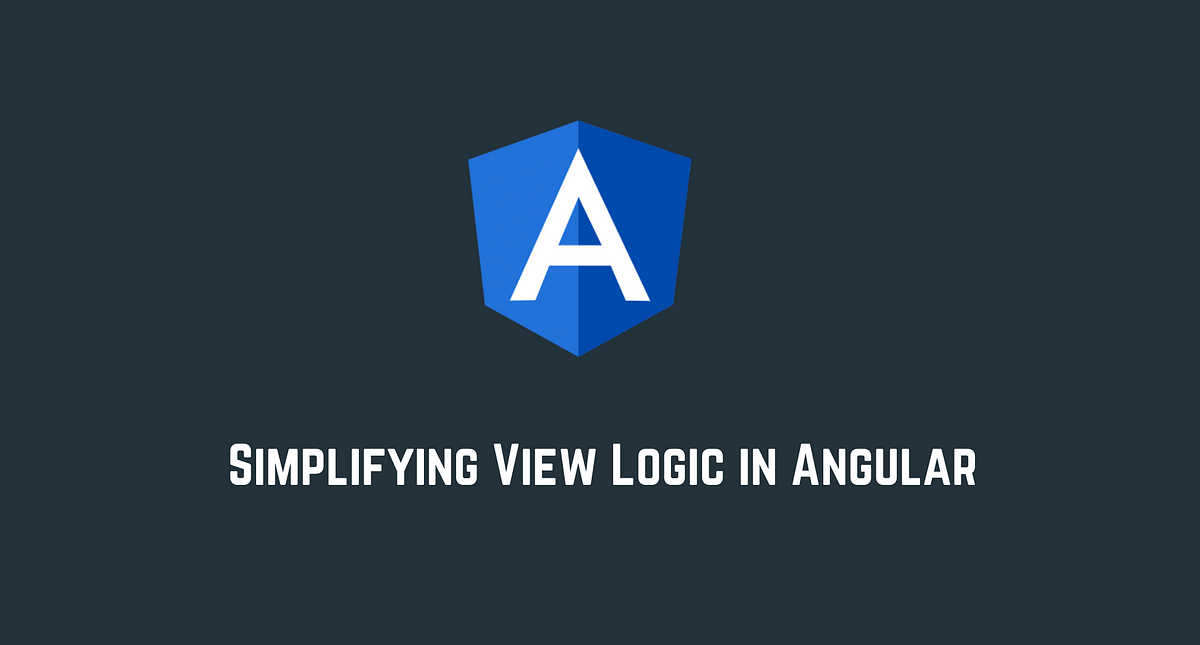
+
Yes, with frameworks like Ionic or NativeScript, you can use Angular to develop cross-platform mobile applications.
Related Terms:
- angular 6 recipe demo
- angular 6 receipe demo
- Recipe book Angular
- Angular 16 projects
- Best Angular portfolio
- Angular 17 projects